a Json String from Flickr.
We can summarise the process as:
A. Create an HMTL file with ng-directives
B. Use $http to connect to read data from a external server
C. Populate a HTML select element with data
D. Display an image from Flickr
For this example you may use Netbeans 8.01
1.Create a new HTML5 project named AJSFlickr and click on Finish
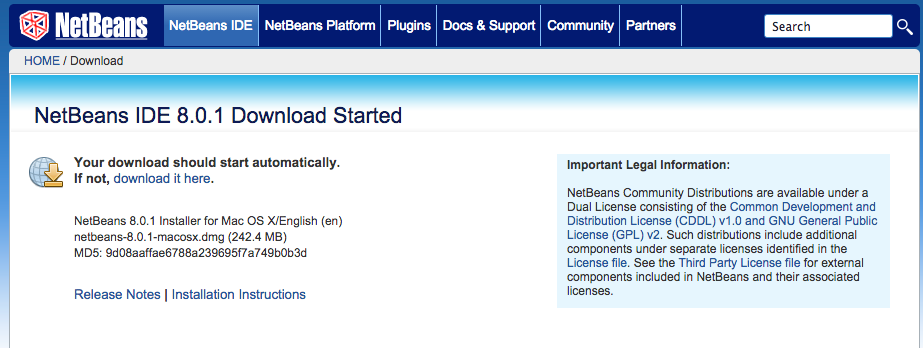
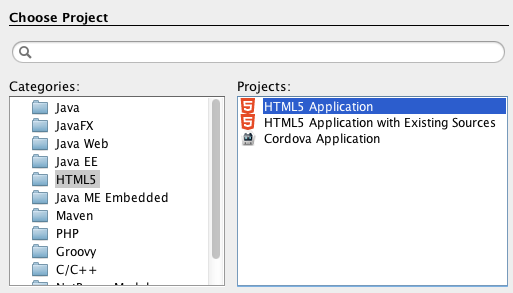
2. Add the following HTML to create the following GUI
<html>
<head>
<title>AJS Example</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<div>
Image description:<input type="text">
<button>Search</button><br/>
<select>
<option>select an option</option>
</select><br/>
<img src="" alt=""/>
</div>
</body>
</html>
3. Lets add angularjs script to the previous code and add the following angularjs directives
data-ng-app:to initialise a AngularJS application
data-ng-model:to bind the HTML elements to dynamic data
data-ng-controller:to create a controller for the elements in the div.
data-ng-click: to perform a click on a button
data-ng-selected:to select an image from the select html element
data-ng-options:to add options for a select
<html data-ng-app="myAJSApp">
<head>
<title>AJS Example</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<div data-ng-controller="AJSFlickrController">
Image description:<input type="text" data-ng-model="description">
<button data-ng-click="searchImage()">Search</button><br/>
<select data-ng-model="selectedImage" data-ng-options="image.title for image in images.photos.photo track by image.id">
<option>select an option</option>
</select><br/>
<img src="https://farm{{selectedImage.farm}}.staticflickr.com/{{selectedImage.server}}/{{selectedImage.id}}_{{selectedImage.secret}}.jpg" alt=""/>
</div>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script>
<script src="myAJSApp.js"></script>
<script src="AJSFlickrController.js"></script>
</body>
</html>
4. Create a javascript file named myAJSApp.js
5. Create a javascript file named AJSFlickrController.js
6. Add the following code to myAJSApp.js to create a module to create a readable application
var app = angular.module("myAJSApp", []);
7. Add the following code to AJSFlickrController.js to use the text entered in the text element and call a function to retrieve the data from
Flickr and populate the select element.
app.config(function ($httpProvider) {
$httpProvider.defaults.useXDomain = true;
delete $httpProvider.defaults.headers.common['X-Requested-With'];
});
app.controller("AJSFlickrController", function ($scope, $http) {
$scope.searchImage = function () {
$http
.get('https://api.flickr.com/services/rest', {
params: {
method: "flickr.photos.search",
api_key: "699982303118892e296bd349c4cc536e",
text: $scope.description,
format: "json"
}
})
.success(function (response, status) {
$scope.images = parseFlickrJson(response);
});
};
});
function parseFlickrJson(jsonstring) {
var data = null;
var jsonFlickrApi = function (d) {
data = d;
}
eval(jsonstring);
return data;
}
8. Open the page in a browser