2. run npm install to install all the required libraries
3. run npm start to start the service. It will open a browser window to test the application
4. open another window
5. run gulp
6. create a new file named first-example.component.ts
7. import the component library
import {Component} from "angular/core"
8. Add a decorator
@Component({
selector:'myTag',
template:'This is a test'
})
9. Add a component class
export class FirstExampleComponent{
}
first-example.component.ts should be like:
import {Component} from "angular2/core"
@Component({
selector:'myTag',
template:'This is a test'
})
export class FirstExampleComponent{
}
10. Open dev/app.components.ts
11. Import FirstExample.component.ts
import {FirstExampleComponent} from "./first-example.component";
12. Add the directives property to @Component with an array of Components that will be used for app.components.ts
directives:[FirstExampleComponent]
13. Modify the template property to show
template: '
app.component.ts should look like:
import {Component} from 'angular2/core';
import {FirstExampleComponent} from "./first-example.component";
@Component({
selector: 'my-app',
template: '
directives:[FirstExampleComponent]
})
export class AppComponent {
}
14. Check the opened browser on step 3:
http://localhost:3000/
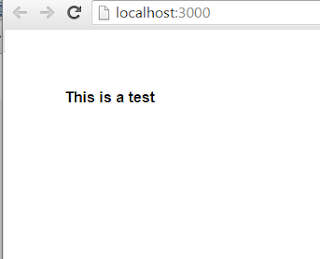