1. Create a new ts file with the following content
import {Component} from "@angular/core"
@Component({
selector:"my-app",
template:"Hello there"
})
export class AppComponent{}
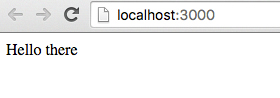
2. Lets try the click event
import {Component} from "@angular/core"
@Component({
selector:"my-app",
template:`
<button (click)="sayHello()">Click</button>
`
})
export class AppComponent{
sayHello(){
alert("Hello there !");
}
}
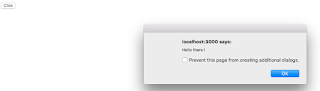
3. Lets try ngFor directive
import {Component} from "@angular/core"
@Component({
selector:"my-app",
template:`
<button (click)="sayHello()">Click</button>
<div *ngFor="#person of people" >
{{person.name}}/{{person.phone}}
</div>
`
})
export class AppComponent{
public people=[{"name":"John Smith","phone":"1234567"},
{"name":"Michael Schumacher","phone":"9876543"},
{"name":"Martin McFly","phone":"443322111"}]
sayHello(){
alert("Hello there !");
}
}
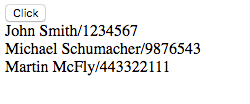
4. Lets add a click event per each one of the divs
import {Component} from "@angular/core"
@Component({
selector:"my-app",
template:`
<button (click)="sayHello()">Click</button>
<div *ngFor="#person of people" (click)="sayHello()">
{{person.name}}/{{person.phone}}
</div>
`
})
export class AppComponent{
public people=[{"name":"John Smith","phone":"1234567"},
{"name":"Michael Schumacher","phone":"9876543"},
{"name":"Martin McFly","phone":"443322111"}]
sayHello(){
alert("Hello there !");
}
}
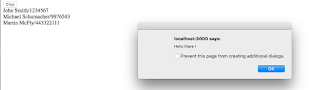
5.We can add edition per each one of the rows
import {Component} from "@angular/core"
@Component({
selector:"my-app",
template:`
<button (click)="sayHello()">Click</button>
<div *ngFor="#person of people" (click)="selectRow(person)">
{{person.name}}/{{person.phone}}
</div>
<input type="text" [(ngModel)]="selectedPerson.name"/>
<input type="text" [(ngModel)]="selectedPerson.phone"/>
`
})
export class AppComponent{
public selectedPerson={};
public people=[{"name":"John Smith","phone":"1234567"},
{"name":"Michael Schumacher","phone":"9876543"},
{"name":"Martin McFly","phone":"443322111"}]
sayHello(){
alert("Hello there !");
}
selectRow(person){
this.selectedPerson=person;
}
}
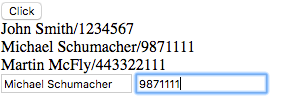
6. Now lets delete an element
import {Component} from "@angular/core"
@Component({
selector:"my-app",
template:`
<div *ngFor="let person of people" (click)="selectRow(person)">
{{person.name}}/{{person.phone}}
<button (click)="deleteRow(person)">Delete</button>
</div>
<input type="text" [(ngModel)]="selectedPerson.name"/>
<input type="text" [(ngModel)]="selectedPerson.phone"/>
`
})
export class AppComponent{
public selectedPerson={};
public people=[{"name":"John Smith","phone":"1234567"},
{"name":"Michael Schumacher","phone":"9876543"},
{"name":"Martin McFly","phone":"443322111"}]
sayHello(){
alert("Hello there !");
}
selectRow(person){
this.selectedPerson=person;
}
deleteRow(person){
this.people.splice(this.people.indexOf(person),1);
}
}
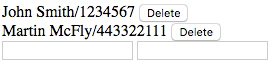
7. Lets add a new record
import {Component} from "@angular/core"
@Component({
selector:"my-app",
template:`
<div *ngFor="let person of people" (click)="selectRow(person)">
{{person.name}}/{{person.phone}}
<button (click)="deleteRow(person)">Delete</button>
</div>
<input type="text" [(ngModel)]="selectedPerson.name"/>
<input type="text" [(ngModel)]="selectedPerson.phone"/>
<button (click)="addPerson()">Add Person
> `
})
export class AppComponent{
public selectedPerson={};
public people=[{"name":"John Smith","phone":"1234567"},
{"name":"Michael Schumacher","phone":"9876543"},
{"name":"Martin McFly","phone":"443322111"}]
sayHello(){
alert("Hello there !");
}
selectRow(person){
this.selectedPerson=person;
}
deleteRow(person){
this.people.splice(this.people.indexOf(person),1);
}
addPerson(){
this.people.push(this.selectedPerson);
}
}
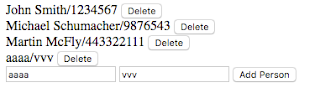
No hay comentarios:
Publicar un comentario